Use GitHub releases to download python versions (#85)
This pull-request improves `setup-python` action to add ability to download specific version of Python on flight if it is not available by default. **Details:** `setup-python` action will download and install specific Python version from GitHub releases ([actions/python-versions](https://github.com/actions/python-versions/releases)) in case the version is not found in the local cache. All versions of Python available for installation are published in [actions/python-versions](https://github.com/actions/python-versions) repository. All available versions are listed in the [version-manifest.json](https://github.com/actions/python-versions/blob/master/versions-manifest.json) file. **Installation time:** - Ubuntu / macOS: 10-20 seconds - Windows: ~ 1 minute (mostly related to fact that we use MSI installer for Python on Windows) Co-authored-by: MaksimZhukov <v-mazhuk@microsoft.com> Co-authored-by: Konrad Pabjan <konradpabjan@github.com> Co-authored-by: Brian Cristante <33549821+brcrista@users.noreply.github.com>
This commit is contained in:
parent
985150d1f6
commit
e5af64b2df
|
@ -0,0 +1,57 @@
|
|||
name: Validate 'setup-python'
|
||||
on:
|
||||
push:
|
||||
pull_request:
|
||||
schedule:
|
||||
- cron: 0 0 * * *
|
||||
|
||||
jobs:
|
||||
default-version:
|
||||
name: Setup default version
|
||||
runs-on: ${{ matrix.os }}
|
||||
strategy:
|
||||
fail-fast: false
|
||||
matrix:
|
||||
os: [macos-latest, windows-latest, ubuntu-16.04, ubuntu-18.04]
|
||||
steps:
|
||||
- name: Checkout
|
||||
uses: actions/checkout@v2
|
||||
|
||||
- name: setup default python
|
||||
uses: ./
|
||||
|
||||
- name: Validate version
|
||||
run: python --version
|
||||
|
||||
- name: Run simple python code
|
||||
run: python -c 'import math; print(math.factorial(5))'
|
||||
|
||||
setup-versions-from-manifest:
|
||||
name: Setup ${{ matrix.python }} ${{ matrix.os }}
|
||||
runs-on: ${{ matrix.os }}
|
||||
strategy:
|
||||
fail-fast: false
|
||||
matrix:
|
||||
os: [macos-latest, windows-latest, ubuntu-16.04, ubuntu-18.04]
|
||||
python: [3.5.4, 3.6.7, 3.7.5, 3.8.1]
|
||||
steps:
|
||||
- name: Checkout
|
||||
uses: actions/checkout@v2
|
||||
|
||||
- name: setup-python ${{ matrix.python }}
|
||||
uses: ./
|
||||
with:
|
||||
python-version: ${{ matrix.python }}
|
||||
|
||||
- name: Validate version
|
||||
run: |
|
||||
$pythonVersion = (python --version)
|
||||
if ("Python ${{ matrix.python }}" -ne "$pythonVersion"){
|
||||
Write-Host "The current version is $pythonVersion; expected version is ${{ matrix.python }}"
|
||||
exit 1
|
||||
}
|
||||
$pythonVersion
|
||||
shell: pwsh
|
||||
|
||||
- name: Run simple code
|
||||
run: python -c 'import math; print(math.factorial(5))'
|
77
README.md
77
README.md
|
@ -6,7 +6,9 @@
|
|||
|
||||
This action sets up a Python environment for use in actions by:
|
||||
|
||||
- optionally installing a version of Python and adding to PATH. Note that this action only uses versions of Python already installed in the cache. The action will fail if no matching versions are found.
|
||||
- optionally installing and adding to PATH a version of Python that is already installed in the tools cache
|
||||
- downloading, installing and adding to PATH an available version of Python from GitHub Releases ([actions/python-versions](https://github.com/actions/python-versions/releases)) if a specific version is not available in the tools cache
|
||||
- failing if a specific version of Python is not preinstalled or available for download
|
||||
- registering problem matchers for error output
|
||||
|
||||
# Usage
|
||||
|
@ -67,13 +69,43 @@ jobs:
|
|||
run: python -c "import sys; print(sys.version)"
|
||||
```
|
||||
|
||||
Download and set up a version of Python that does not come preinstalled on an image:
|
||||
```yaml
|
||||
jobs:
|
||||
build:
|
||||
runs-on: ubuntu-latest
|
||||
strategy:
|
||||
# in this example, there is a newer version already installed, 3.7.7, so the older version will be downloaded
|
||||
python-version: [3.5, 3.6, 3.7.4, 3.8]
|
||||
steps:
|
||||
- uses: actions/checkout@v2
|
||||
- uses: actions/setup-python@v1
|
||||
with:
|
||||
python-version: ${{ matrix.python }}
|
||||
- run: python my_script.py
|
||||
|
||||
```
|
||||
|
||||
# Getting started with Python + Actions
|
||||
|
||||
Check out our detailed guide on using [Python with GitHub Actions](https://help.github.com/en/actions/automating-your-workflow-with-github-actions/using-python-with-github-actions).
|
||||
|
||||
# Available versions of Python
|
||||
|
||||
`setup-python` is able to configure Python from two sources:
|
||||
|
||||
- Preinstalled versions of Python in the tools cache on GitHub-hosted runners
|
||||
- For detailed information regarding the available versions of Python that are installed see [Software installed on GitHub-hosted runners](https://help.github.com/en/actions/automating-your-workflow-with-github-actions/software-installed-on-github-hosted-runners).
|
||||
- For every minor version of Python, expect only the latest patch to be preinstalled.
|
||||
- If `3.8.1` is installed for example, and `3.8.2` is released, expect `3.8.1` to be removed and replaced by `3.8.2` in the tools cache.
|
||||
- If the exact patch version doesn't matter to you, specifying just the major and minor version will get you the latest preinstalled patch version. In the previous example, the version spec `3.8` will use the `3.8.2` Python version found in the cache.
|
||||
- Downloadable Python versions from GitHub Releases ([actions/python-versions](https://github.com/actions/python-versions/releases))
|
||||
- All available versions are listed in the [version-manifest.json](https://github.com/actions/python-versions/blob/master/versions-manifest.json) file.
|
||||
- If there is a specific version of Python that is not available, you can open an issue in the `python-versions` repository.
|
||||
|
||||
# Hosted Tool Cache
|
||||
|
||||
GitHub hosted runners have a tools cache that comes with Python + PyPy already installed. This tools cache helps speed up runs and tool setup by not requiring any new downloads. There is an environment variable called `RUNNER_TOOL_CACHE` on each runner that describes the location of this tools cache and there is where you will find Python and PyPy installed. `setup-python` works by taking a specific version of Python or PyPy in this tools cache and adding it to PATH.
|
||||
GitHub hosted runners have a tools cache that comes with a few versions of Python + PyPy already installed. This tools cache helps speed up runs and tool setup by not requiring any new downloads. There is an environment variable called `RUNNER_TOOL_CACHE` on each runner that describes the location of this tools cache and there is where you will find Python and PyPy installed. `setup-python` works by taking a specific version of Python or PyPy in this tools cache and adding it to PATH.
|
||||
|
||||
|| Location |
|
||||
|------|-------|
|
||||
|
@ -85,30 +117,39 @@ GitHub virtual environments are setup in [actions/virtual-environments](https://
|
|||
- [Tools cache setup for Ubuntu](https://github.com/actions/virtual-environments/blob/master/images/linux/scripts/installers/hosted-tool-cache.sh)
|
||||
- [Tools cache setup for Windows](https://github.com/actions/virtual-environments/blob/master/images/win/scripts/Installers/Download-ToolCache.ps1)
|
||||
|
||||
# Specifying a Python version
|
||||
|
||||
If there is a specific version of Python that you need and you don't want to worry about any potential breaking changes due to patch updates (going from `3.7.5` to `3.7.6` for example), you should specify the exact major, minor, and patch version (such as `3.7.5`)
|
||||
- The only downside to this is that set up will take a little longer since the exact version will have to be downloaded if the exact version is not already installed on the runner due to more recent versions.
|
||||
- MSI installers are used on Windows for this, so runs will take a little longer to set up vs Mac and Linux.
|
||||
|
||||
You should specify only a major and minor version if you are okay with the most recent patch version being used.
|
||||
- There will be a single patch version already installed on each runner for every minor version of Python that is supported.
|
||||
- The patch version that will be preinstalled, will generally be the latest and every time there is a new patch released, the older version that is preinstalled will be replaced.
|
||||
- Using the most recent patch version will result in a very quick setup since no downloads will be required since a locally installed version Python on the runner will be used.
|
||||
|
||||
# Using `setup-python` with a self hosted runner
|
||||
|
||||
If you would like to use `setup-python` on a self-hosted runner, you will need to download all versions of Python & PyPy that you would like and setup a similar tools cache locally for your runner.
|
||||
If you would like to use `setup-python` and a self-hosted runner, there isn't much that you need to do. When `setup-python` is run for the first time with a version of Python that it doesn't have, it will download the appropriate version, and set up the tools cache on your machine. Any subsequent runs will use the Python versions that were previously downloaded.
|
||||
|
||||
- Create an global environment variable called `AGENT_TOOLSDIRECTORY` that will point to the root directory of where you want the tools installed. The env variable is preferrably global as it must be set in the shell that will install the tools cache, along with the shell that the runner will be using.
|
||||
- This env variable is used internally by the runner to set the `RUNNER_TOOL_CACHE` env variable
|
||||
- Example for Administrator Powershell: `[System.Environment]::SetEnvironmentVariable("AGENT_TOOLSDIRECTORY", "C:\hostedtoolcache\windows", [System.EnvironmentVariableTarget]::Machine)` (restart the shell afterwards)
|
||||
- Download the appropriate NPM packages from the [GitHub Actions NPM registry](https://github.com/orgs/actions/packages)
|
||||
- Make sure to have `npm` installed, and then [configure npm for use with GitHub packages](https://help.github.com/en/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages#authenticating-to-github-package-registry)
|
||||
- Create an empty npm project for easier installation (`npm init`) in the tools cache directory. You can delete `package.json`, `package.lock.json` and `node_modules` after all tools get installed
|
||||
- Before downloading a specific package, create an empty folder for the version of Python/PyPY that is being installed. If downloading Python 3.6.8 for example, create `C:\hostedtoolcache\windows\Python\3.6.8`
|
||||
- Once configured, download a specific package by calling `npm install`. Note (if downloading a PyPy package on Windows, you will need 7zip installed along with `7z.exe` added to your PATH)
|
||||
- Each NPM package has multiple versions that determine the version of Python or PyPy that should be installed.
|
||||
- `npm install @actions/toolcache-python-windows-x64@3.7.61579791175` for example installs Python 3.7.6 while `npm install @actions/toolcache-python-windows-x64@3.6.81579791177` installs Python 3.6.8
|
||||
- You can browse and find all available versions of a package by searching the GitHub Actions NPM registry
|
||||
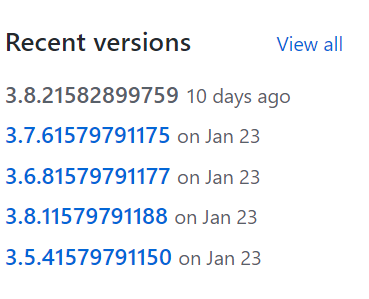
|
||||
A few things to look out for when `setup-python` is first setting up the tools cache
|
||||
- If using Windows, your runner needs to be running as an administrator so that the appropriate directories and files can be setup. On Linux and Mac, you also need to be running with elevated permissions
|
||||
- On Windows, you need `7zip` installed and added to your `PATH` so that files can be extracted properly during setup
|
||||
- MSI installers are used when setting up Python on Windows. A word of caution as MSI installers update registry settings
|
||||
- The 3.8 MSI installer for Windows will not let you install another 3.8 version of Python. If `setup-python` fails for a 3.8 version of Python, make sure any previously installed versions are removed by going to "Apps & Features" in the Settings app.
|
||||
|
||||
# Using Python without `setup-python`
|
||||
|
||||
`setup-python` helps keep your dependencies explicit and ensures consistent behavior between different runners. If you use `python` in a shell on a GitHub hosted runner without `setup-python` it will default to whatever is in PATH. The default version of Python in PATH vary between runners and can change unexpectedly so we recommend you always use `setup-python`.
|
||||
|
||||
# Available versions of Python
|
||||
# Need to open an issue?
|
||||
|
||||
For detailed information regarding the available versions of Python that are installed see [Software installed on GitHub-hosted runners](https://help.github.com/en/actions/automating-your-workflow-with-github-actions/software-installed-on-github-hosted-runners)
|
||||
Python versions available for `setup-python` can be found in the [actions/python-versions](https://github.com/actions/python-versions) repository. You can see the build scripts, configurations, and everything that is used. You should open an issue in the `python-versions` repository if:
|
||||
- something might be compiled incorrectly
|
||||
- certain modules might be missing
|
||||
- there is a version of Python that you would like that is currently not available
|
||||
|
||||
Any remaining issues can be filed in this repository
|
||||
|
||||
# License
|
||||
|
||||
|
@ -116,4 +157,4 @@ The scripts and documentation in this project are released under the [MIT Licens
|
|||
|
||||
# Contributions
|
||||
|
||||
Contributions are welcome! See [Contributor's Guide](docs/contributors.md)
|
||||
Contributions are welcome! See our [Contributor's Guide](docs/contributors.md)
|
||||
|
|
|
@ -0,0 +1,13 @@
|
|||
{
|
||||
"version": "1.2.3",
|
||||
"stable": true,
|
||||
"release_url": "https://github.com/actions/sometool/releases/tag/1.2.3-20200402.6",
|
||||
"files": [
|
||||
{
|
||||
"filename": "sometool-1.2.3-linux-x64.tar.gz",
|
||||
"arch": "x64",
|
||||
"platform": "linux",
|
||||
"download_url": "https://github.com/actions/sometool/releases/tag/1.2.3-20200402.6/sometool-1.2.3-linux-x64.tar.gz"
|
||||
}
|
||||
]
|
||||
}
|
|
@ -18,19 +18,48 @@ const tempDir = path.join(
|
|||
process.env['RUNNER_TOOL_CACHE'] = toolDir;
|
||||
process.env['RUNNER_TEMP'] = tempDir;
|
||||
|
||||
import * as tc from '@actions/tool-cache';
|
||||
import * as finder from '../src/find-python';
|
||||
import * as installer from '../src/install-python';
|
||||
|
||||
const pythonRelease = require('./data/python-release.json');
|
||||
|
||||
describe('Finder tests', () => {
|
||||
afterEach(() => {
|
||||
jest.resetAllMocks();
|
||||
jest.clearAllMocks();
|
||||
});
|
||||
|
||||
it('Finds Python if it is installed', async () => {
|
||||
const pythonDir: string = path.join(toolDir, 'Python', '3.0.0', 'x64');
|
||||
await io.mkdirP(pythonDir);
|
||||
fs.writeFileSync(`${pythonDir}.complete`, 'hello');
|
||||
// This will throw if it doesn't find it in the cache (because no such version exists)
|
||||
// This will throw if it doesn't find it in the cache and in the manifest (because no such version exists)
|
||||
await finder.findPythonVersion('3.x', 'x64');
|
||||
});
|
||||
|
||||
it('Finds Python if it is not installed, but exists in the manifest', async () => {
|
||||
const findSpy: jest.SpyInstance = jest.spyOn(
|
||||
installer,
|
||||
'findReleaseFromManifest'
|
||||
);
|
||||
findSpy.mockImplementation(() => <tc.IToolRelease>pythonRelease);
|
||||
|
||||
const installSpy: jest.SpyInstance = jest.spyOn(
|
||||
installer,
|
||||
'installCpythonFromRelease'
|
||||
);
|
||||
installSpy.mockImplementation(async () => {
|
||||
const pythonDir: string = path.join(toolDir, 'Python', '1.2.3', 'x64');
|
||||
await io.mkdirP(pythonDir);
|
||||
fs.writeFileSync(`${pythonDir}.complete`, 'hello');
|
||||
});
|
||||
// This will throw if it doesn't find it in the cache and in the manifest (because no such version exists)
|
||||
await finder.findPythonVersion('1.2.3', 'x64');
|
||||
});
|
||||
|
||||
it('Errors if Python is not installed', async () => {
|
||||
// This will throw if it doesn't find it in the cache (because no such version exists)
|
||||
// This will throw if it doesn't find it in the cache and in the manifest (because no such version exists)
|
||||
let thrown = false;
|
||||
try {
|
||||
await finder.findPythonVersion('3.300000', 'x64');
|
||||
|
|
|
@ -8,7 +8,9 @@ inputs:
|
|||
default: '3.x'
|
||||
architecture:
|
||||
description: 'The target architecture (x86, x64) of the Python interpreter.'
|
||||
default: 'x64'
|
||||
token:
|
||||
description: Used to pull python distributions from actions/python-versions. Since there's a default, this is typically not supplied by the user.
|
||||
default: ${{ github.token }}
|
||||
outputs:
|
||||
python-version:
|
||||
description: "The installed python version. Useful when given a version range as input."
|
||||
|
|
File diff suppressed because it is too large
Load Diff
|
@ -19,9 +19,9 @@
|
|||
}
|
||||
},
|
||||
"@actions/http-client": {
|
||||
"version": "1.0.6",
|
||||
"resolved": "https://registry.npmjs.org/@actions/http-client/-/http-client-1.0.6.tgz",
|
||||
"integrity": "sha512-LGmio4w98UyGX33b/W6V6Nx/sQHRXZ859YlMkn36wPsXPB82u8xTVlA/Dq2DXrm6lEq9RVmisRJa1c+HETAIJA==",
|
||||
"version": "1.0.8",
|
||||
"resolved": "https://registry.npmjs.org/@actions/http-client/-/http-client-1.0.8.tgz",
|
||||
"integrity": "sha512-G4JjJ6f9Hb3Zvejj+ewLLKLf99ZC+9v+yCxoYf9vSyH+WkzPLB2LuUtRMGNkooMqdugGBFStIKXOuvH1W+EctA==",
|
||||
"dev": true,
|
||||
"requires": {
|
||||
"tunnel": "0.0.6"
|
||||
|
@ -34,14 +34,14 @@
|
|||
"dev": true
|
||||
},
|
||||
"@actions/tool-cache": {
|
||||
"version": "1.3.3",
|
||||
"resolved": "https://registry.npmjs.org/@actions/tool-cache/-/tool-cache-1.3.3.tgz",
|
||||
"integrity": "sha512-AFVyTLcIxusDVI1gMhbZW8m/On7YNJG+xYaxorV+qic+f7lO7h37aT2mfzxqAq7mwHxtP1YlVFNrXe9QDf/bPg==",
|
||||
"version": "1.5.2",
|
||||
"resolved": "https://registry.npmjs.org/@actions/tool-cache/-/tool-cache-1.5.2.tgz",
|
||||
"integrity": "sha512-40A1St0GEo+QvHV1YRjStEoQcKKMaip+zNXPgGHcjYXCdZ7cl1LGlwOpsVVqwk+6ue/shFTS76KC1A02mVVCQA==",
|
||||
"dev": true,
|
||||
"requires": {
|
||||
"@actions/core": "^1.2.0",
|
||||
"@actions/exec": "^1.0.0",
|
||||
"@actions/http-client": "^1.0.3",
|
||||
"@actions/http-client": "^1.0.8",
|
||||
"@actions/io": "^1.0.1",
|
||||
"semver": "^6.1.0",
|
||||
"uuid": "^3.3.2"
|
||||
|
|
|
@ -28,7 +28,7 @@
|
|||
},
|
||||
"devDependencies": {
|
||||
"@actions/io": "^1.0.2",
|
||||
"@actions/tool-cache": "^1.3.3",
|
||||
"@actions/tool-cache": "^1.5.2",
|
||||
"@types/jest": "^25.1.4",
|
||||
"@types/node": "^12.12.31",
|
||||
"@types/semver": "^7.1.0",
|
||||
|
|
|
@ -3,22 +3,7 @@ import * as path from 'path';
|
|||
|
||||
import * as semver from 'semver';
|
||||
|
||||
let cacheDirectory = process.env['RUNNER_TOOLSDIRECTORY'] || '';
|
||||
|
||||
if (!cacheDirectory) {
|
||||
let baseLocation;
|
||||
if (process.platform === 'win32') {
|
||||
// On windows use the USERPROFILE env variable
|
||||
baseLocation = process.env['USERPROFILE'] || 'C:\\';
|
||||
} else {
|
||||
if (process.platform === 'darwin') {
|
||||
baseLocation = '/Users';
|
||||
} else {
|
||||
baseLocation = '/home';
|
||||
}
|
||||
}
|
||||
cacheDirectory = path.join(baseLocation, 'actions', 'cache');
|
||||
}
|
||||
import * as installer from './install-python';
|
||||
|
||||
import * as core from '@actions/core';
|
||||
import * as tc from '@actions/tool-cache';
|
||||
|
@ -92,29 +77,33 @@ async function useCpythonVersion(
|
|||
const semanticVersionSpec = pythonVersionToSemantic(desugaredVersionSpec);
|
||||
core.debug(`Semantic version spec of ${version} is ${semanticVersionSpec}`);
|
||||
|
||||
const installDir: string | null = tc.find(
|
||||
let installDir: string | null = tc.find(
|
||||
'Python',
|
||||
semanticVersionSpec,
|
||||
architecture
|
||||
);
|
||||
if (!installDir) {
|
||||
// Fail and list available versions
|
||||
const x86Versions = tc
|
||||
.findAllVersions('Python', 'x86')
|
||||
.map(s => `${s} (x86)`)
|
||||
.join(os.EOL);
|
||||
core.info(
|
||||
`Version ${semanticVersionSpec} was not found in the local cache`
|
||||
);
|
||||
const foundRelease = await installer.findReleaseFromManifest(
|
||||
semanticVersionSpec,
|
||||
architecture
|
||||
);
|
||||
|
||||
const x64Versions = tc
|
||||
.findAllVersions('Python', 'x64')
|
||||
.map(s => `${s} (x64)`)
|
||||
.join(os.EOL);
|
||||
if (foundRelease && foundRelease.files && foundRelease.files.length > 0) {
|
||||
core.info(`Version ${semanticVersionSpec} is available for downloading`);
|
||||
await installer.installCpythonFromRelease(foundRelease);
|
||||
|
||||
installDir = tc.find('Python', semanticVersionSpec, architecture);
|
||||
}
|
||||
}
|
||||
|
||||
if (!installDir) {
|
||||
throw new Error(
|
||||
[
|
||||
`Version ${version} with arch ${architecture} not found`,
|
||||
'Available versions:',
|
||||
x86Versions,
|
||||
x64Versions
|
||||
`The list of all available versions can be found here: ${installer.MANIFEST_URL}`
|
||||
].join(os.EOL)
|
||||
);
|
||||
}
|
||||
|
|
|
@ -0,0 +1,65 @@
|
|||
import * as path from 'path';
|
||||
import * as core from '@actions/core';
|
||||
import * as tc from '@actions/tool-cache';
|
||||
import * as exec from '@actions/exec';
|
||||
import {ExecOptions} from '@actions/exec/lib/interfaces';
|
||||
|
||||
const AUTH_TOKEN = core.getInput('token');
|
||||
const MANIFEST_REPO_OWNER = 'actions';
|
||||
const MANIFEST_REPO_NAME = 'python-versions';
|
||||
export const MANIFEST_URL = `https://raw.githubusercontent.com/${MANIFEST_REPO_OWNER}/${MANIFEST_REPO_NAME}/master/versions-manifest.json`;
|
||||
|
||||
const IS_WINDOWS = process.platform === 'win32';
|
||||
|
||||
export async function findReleaseFromManifest(
|
||||
semanticVersionSpec: string,
|
||||
architecture: string
|
||||
): Promise<tc.IToolRelease | undefined> {
|
||||
const manifest: tc.IToolRelease[] = await tc.getManifestFromRepo(
|
||||
MANIFEST_REPO_OWNER,
|
||||
MANIFEST_REPO_NAME,
|
||||
AUTH_TOKEN
|
||||
);
|
||||
return await tc.findFromManifest(
|
||||
semanticVersionSpec,
|
||||
true,
|
||||
manifest,
|
||||
architecture
|
||||
);
|
||||
}
|
||||
|
||||
async function installPython(workingDirectory: string) {
|
||||
const options: ExecOptions = {
|
||||
cwd: workingDirectory,
|
||||
silent: true,
|
||||
listeners: {
|
||||
stdout: (data: Buffer) => {
|
||||
core.debug(data.toString().trim());
|
||||
}
|
||||
}
|
||||
};
|
||||
|
||||
if (IS_WINDOWS) {
|
||||
await exec.exec('powershell', ['./setup.ps1'], options);
|
||||
} else {
|
||||
await exec.exec('bash', ['./setup.sh'], options);
|
||||
}
|
||||
}
|
||||
|
||||
export async function installCpythonFromRelease(release: tc.IToolRelease) {
|
||||
const downloadUrl = release.files[0].download_url;
|
||||
|
||||
core.info(`Download from "${downloadUrl}"`);
|
||||
const pythonPath = await tc.downloadTool(downloadUrl, undefined, AUTH_TOKEN);
|
||||
const fileName = path.basename(pythonPath, '.zip');
|
||||
core.info('Extract downloaded archive');
|
||||
let pythonExtractedFolder;
|
||||
if (IS_WINDOWS) {
|
||||
pythonExtractedFolder = await tc.extractZip(pythonPath, `./${fileName}`);
|
||||
} else {
|
||||
pythonExtractedFolder = await tc.extractTar(pythonPath, `./${fileName}`);
|
||||
}
|
||||
|
||||
core.info('Execute installation script');
|
||||
await installPython(pythonExtractedFolder);
|
||||
}
|
|
@ -1,12 +1,13 @@
|
|||
import * as core from '@actions/core';
|
||||
import * as finder from './find-python';
|
||||
import * as path from 'path';
|
||||
import * as os from 'os';
|
||||
|
||||
async function run() {
|
||||
try {
|
||||
let version = core.getInput('python-version');
|
||||
if (version) {
|
||||
const arch: string = core.getInput('architecture', {required: true});
|
||||
const arch: string = core.getInput('architecture') || os.arch();
|
||||
const installed = await finder.findPythonVersion(version, arch);
|
||||
core.info(`Successfully setup ${installed.impl} (${installed.version})`);
|
||||
}
|
||||
|
|
Loading…
Reference in New Issue